Runtime Script
The Runtime Script is used to create program code and run it trigger-based. This can be used, for example, to query signal values, set target values based on events, implement a batch start or stop, or transfer a camera image to the archive. It is also possible to use a script to check whether a camera image is available in the archive within a certain period of time.
The "Runtime Script" configuration unit can only be used if the SCADA license includes the "Script Module" module.
A new object can be created directly via the plus. If you want to edit or search for an object, click on the button (the area around the plus) to get a list of all existing objects. There you can select the object that should be edited.
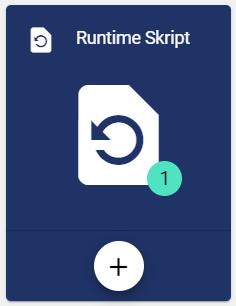
Basic configuration
The name of the object must be assigned. Additionally, a description text can be added optionally.
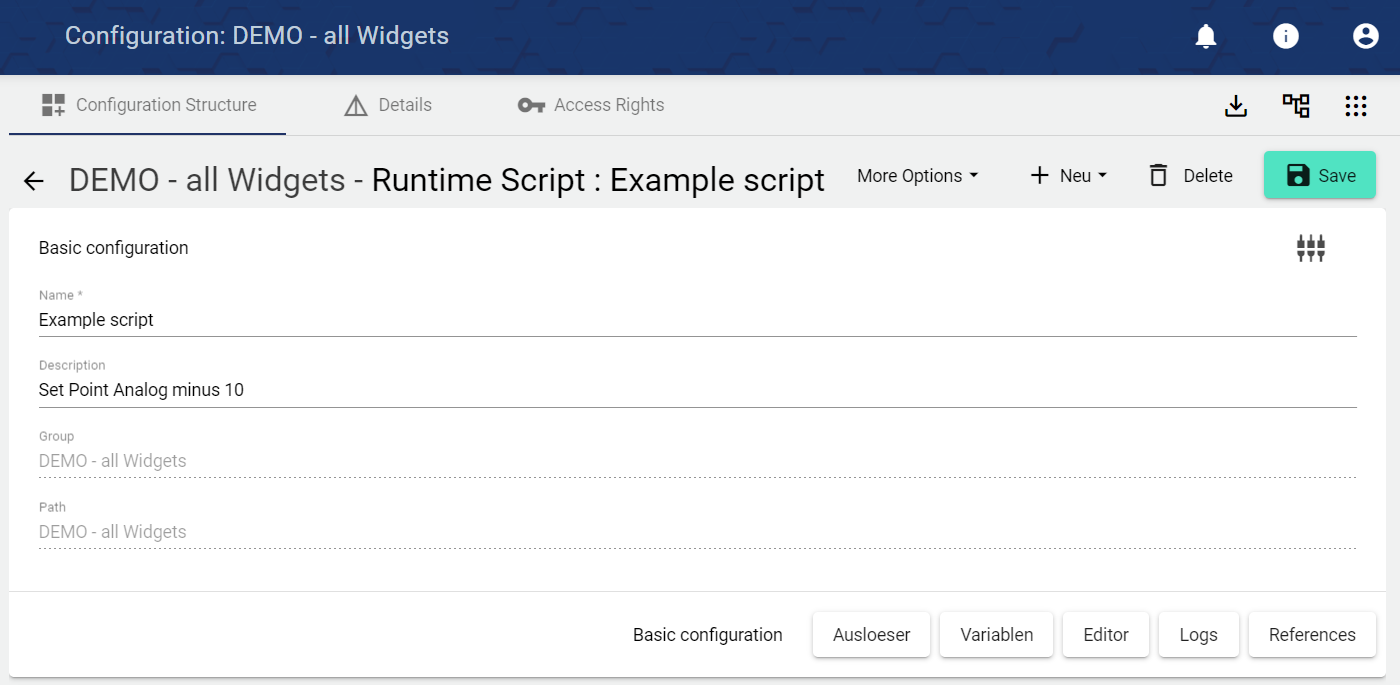
Triggers
The trigger specifies when or in which cycle the following script is to be executed. There are several options available. Enclosed is an example with all available options.
Typ | Wert | Aktion | Beschreibung |
---|---|---|---|
Cyclic Trigger | Integer | - | Periodic trigger |
Event Trigger |
| Event based trigger | |
Condition Trigger |
| Signal condition as trigger | |
Batch Trigger |
| Batch start or stop as trigger |
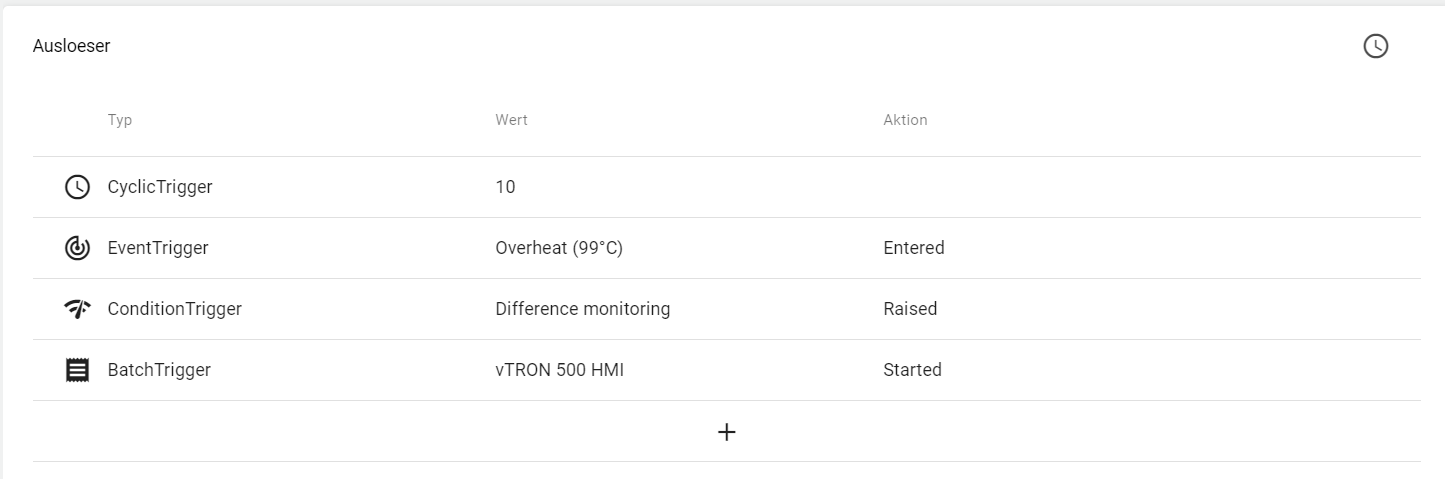
Variables
To make the Runtime Script usable for object orientation or to clearly display the variables in the script, so-called “static variables” can be created outside the script, which can then be used within the script:

The variable SignalID can then be used like this in the script or editor. For more information, see the Editor chapter.

Editor
The script editor is used to enable the actual program flow. The programming language is JavaScript. The functionality of JavaScript (ES2022) is mostly available, a detailed list of what is supported can be found on: https://github.com/sebastienros/jint.
The script can be executed manually via the "Test" and "Execute" buttons. These manual actions are recorded and logged in the "History" tab. Clicking on "Test" creates a "Dry Run" in the history. No setpoints are set, no batches are started or stopped, and no camera images are stored in the archive. Under History, however, the user can view calculated values or script feedback. A click on "Execute" executes the script completely.
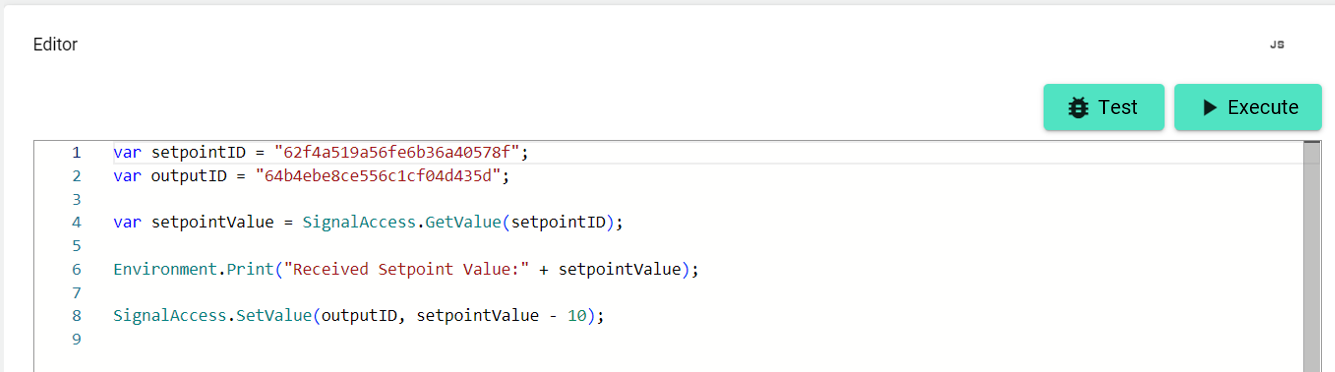
To interact with the JUMO smartWARE SCADA application in the script, some functions and namespaces are provided. To call a function on a namespace, write:
{Namespace}.{Function call}
The following are the namespaces and their functions.
Italicized expressions in curly brackets are used as placeholders, i.e. in a real use case they must be replaced by variables or values. {SignalID}
SignalAccess
The SignalAccess namespace provides functions for reading values from signals and setting setpoints.
With the function SignalAccess.GetValue({SignalID}): number | string the current live value of the signal specified by the parameter can be read out. The value is returned as a number or string. If the signal does not exist or the parameter {SignalID} is empty, zero is returned.
SignalAccess.GetValue({SignalID}): number | string
With the function GetValueWithTimestamp({SignalID}): {Value: number | string, Timestamp:
Date} the current live value can be read out together with the associated time stamp of the signal specified via the parameter. If the signal does not exist or the {SignalID} parameter is empty, zero is returned.
SignalAccess.GetValueWithTimestamp({SignalID}): {Value: number | string, Timestamp:
Date}
The SignalAccess.SetValue({SignalID}, {Value}): void function can be used to set the value of a signal (output), i.e. the value specified in the second parameter {Value} is sent to the gateway or in case of a virtual signal (signal without data source and data connection) is generated as live value. The signal is specified via the first parameter {SignalID}.
SignalAccess.SetValue({SignalID}, {Value}): void
Virtual signals without data source and data connection are currently not stored historically.
BatchAccess
The BatchAccess namespace provides functions to start/stop batches and query active batches.
The function BatchAccess.GetRunningBatches({BatchDefinitionID}): Batch[] can be used to query the active running batches, the batch definition specified by the {BatchDefinitionID} parameter.
BatchAccess.GetRunningBatches({BatchDefinitionID}): Batch[]
The function BachAccess.StartBatch({BatchDefinitionId}, {MetadataValues}): Batch can be used to start new batches. The parameter {BatchDefinitionID} specifies the desired batch definition. The header data is transferred via the second parameter {MetadataValues}. The exact name of the header data field must be taken from the batch definition. Subsequently, the corresponding value is specified by colon. For example {Product type: 300} or {Product name: Product1}. If several header data are set, they can be listed one after the other by comma {Product type: 300, Product name: "Wiener sausages"}.
BachAccess.StartBatch({BatchDefinitionId}, {MetadataValues}): Batch
The function BachAccess.StopBatch({BatchDefinitionId}, {BatchId}, {MetadataValues}): Batch can be used to stop running batches. The {BatchDefinitionID} parameter specifies the current batch definition. The parameter {BatchId} specifies the respective batch. The header data is transferred via the third parameter {MetadataValues}. Here the exact name of the header data field must be taken from the batch definition. Subsequently, the corresponding value is specified by colon. For example {Product type: 300} or {Product name: Product1}. If several header data are set, they can be listed one after the other by comma {Product type: 300, Product name: "Wiener sausages"}.
BachAccess.StopBatch({BatchDefinitionId}, {BatchId}, {MetadataValues}): Batch
CameraAccess
The CameraAccess namespace provides functions to create and retrieve archive images from cameras.
With CameraAccess.AnyArchiveImage({CameraID}, {From}, {Till}): boolean function can be used to check whether an archive image exists or not. The parameter {CameraID} specified the respective camera. The parameter {From}, {Till} specified the time period to query if an archive image exists.
CameraAccess.AnyArchiveImage({CameraID}, {From}, {Till}): boolean
The CameraAccess.TakeArchiveImage({CameraId}): void function can be used to take an archive image. The {CameraID} parameter specified the respective camera.
CameraAccess.TakeArchiveImage({CameraId}): void
Environment
The Environment namespace provides general utility functions to simplify scripting and generate log entries in the History.
The Environment.Print({Message}) function can be used to write messages of any kind as log entries in the history of the script to make them easier to debug. However, the values must be serializable.
Environment.Print({Message})
The Environment.GetCurrentUtcDateTime() function can be used to retrieve the current time in UTC.
Environment.GetCurrentUtcDateTime()
Save status across multiple script executions
There may be times when you want to persistently store certain variables or values {message} to use them the next time you run the script. This is made possible by the objectScriptResources[{Message}].
ScriptResources[{Message}]
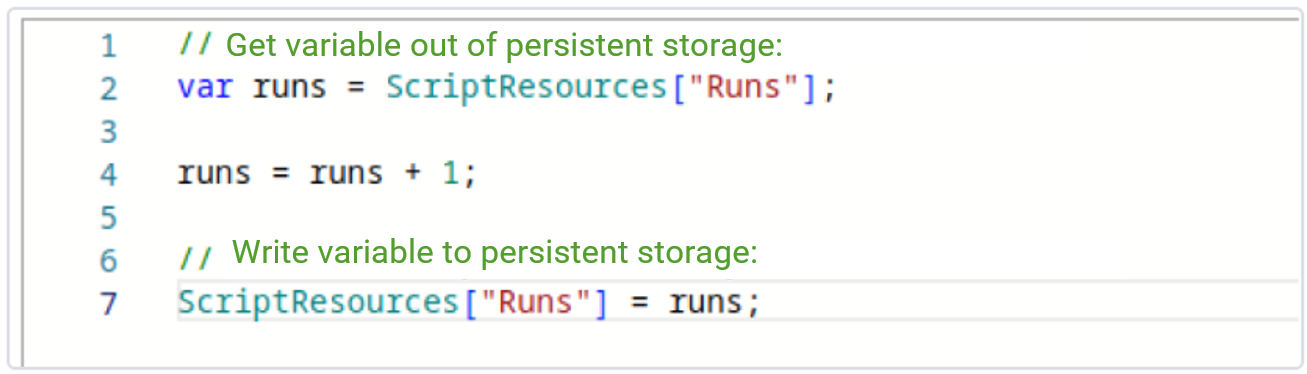
History
Each triggering of the script is stored in the history. A manual trigger via the "Test" or "Execute" button is recorded as a manual entry. A "Test" creates a "Dry Run" in which no actual script actions are executed.
The log can be viewed via the plus symbol on the right. Here actions of the script are listed and calculated or queried or set values can be viewed.
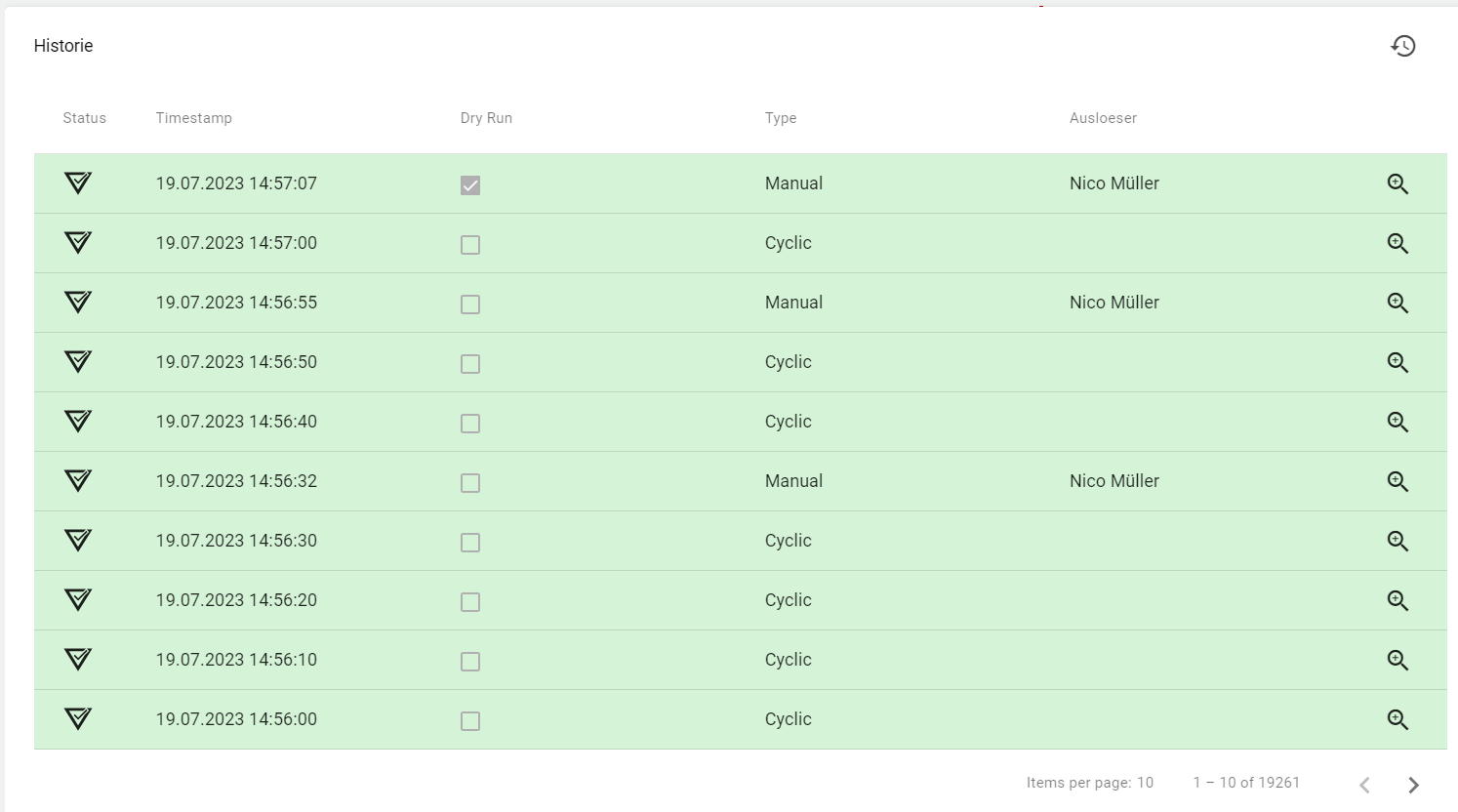
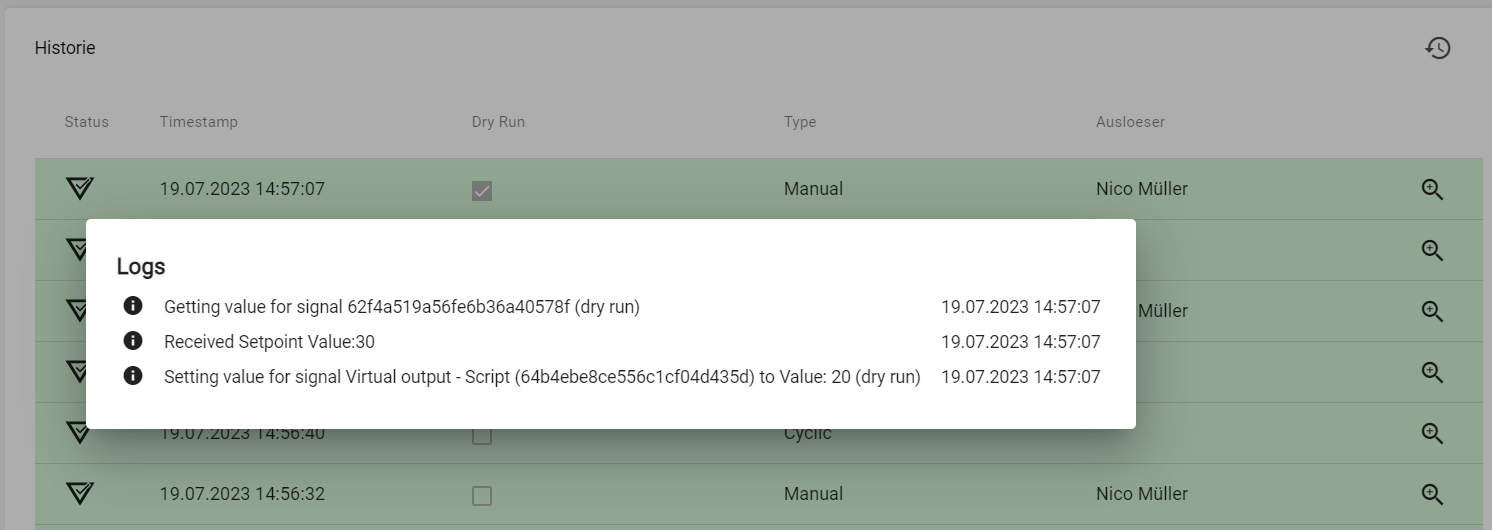
References
All objects that have references with the object appear in the references.
AuditLog
The AuditLog is a change log that transparently shows how the object was changed. For some objects, it also shows which target values were set by a specific person. Power users can use the button on the right-hand side to get a detailed view of exactly what has been changed in the configuration.
The AuditLog can be used to quickly trace what happened to the object last. It corresponds to an extract from the Control Operation Archive.